Building Real-Time Applications with AWS AppSync
- Ashish Tiwari
- Aug 17, 2024
- 4 min read
Updated: 4 days ago
In today’s fast-paced digital world, real-time applications are essential for providing instant updates and seamless user experiences. AWS AppSync is a managed service that makes it easy to build scalable, real-time applications on AWS. In this blog, we'll explore the essentials of AWS AppSync and provide a step-by-step guide to building your own real-time application. We'll also include architecture diagrams, real-world use cases, and best practices to help you get started.
What is AWS AppSync?
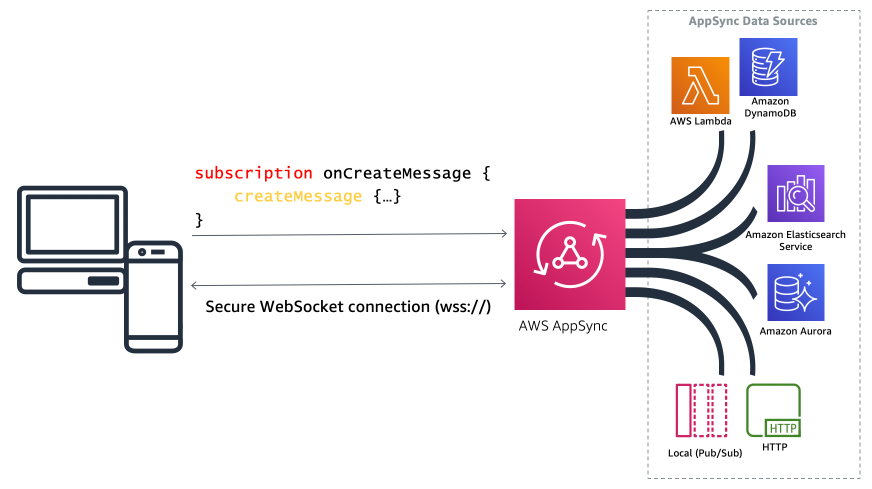
AWS AppSync is a fully managed service that simplifies the development of GraphQL APIs by integrating with AWS services like DynamoDB, Lambda, and Elasticsearch. It enables you to build real-time applications with features like offline data synchronization and automatic conflict resolution, ensuring a smooth user experience even when network connectivity is unreliable.
Step-by-Step Guide to Building a Real-Time Application with AWS AppSync
Step 1: Setting Up Your AWS Environment
Before you start building your application, you need to set up your AWS environment:
AWS Account: If you don't already have an AWS account, sign up at aws.amazon.com.
IAM Roles: Create IAM roles with appropriate permissions for accessing AWS services like DynamoDB and Lambda.
Step 2: Creating a GraphQL API with AppSync
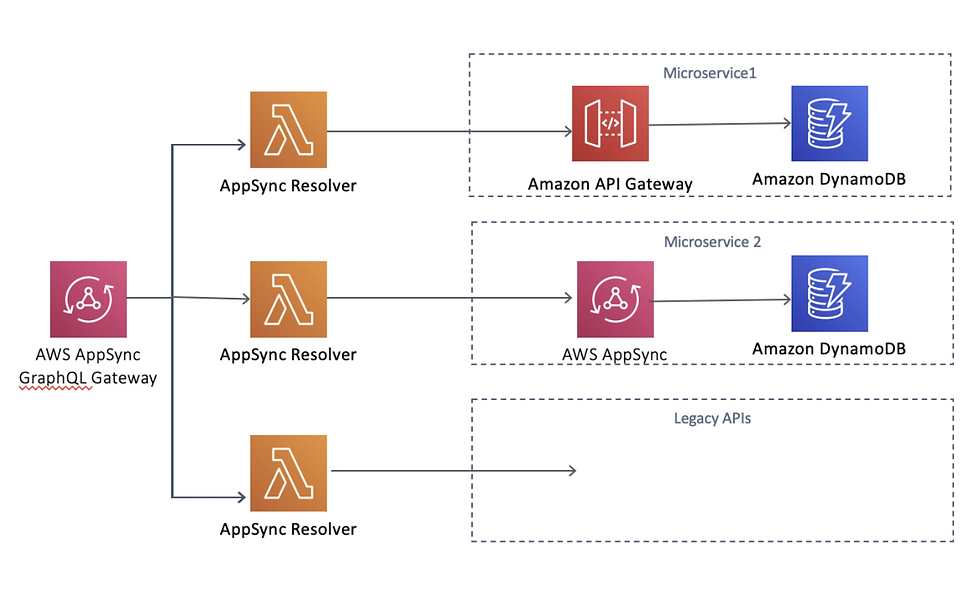
Navigate to the AppSync Console: In the AWS Management Console, navigate to the AppSync console.
Create API: Click on "Create API," choose the "Start from scratch" option, and provide a name for your API.
Schema Definition: Define your GraphQL schema. For example, a simple chat application schema might look like this:
type Message {
id: ID!
content: String!
timestamp: String!
}
type Query {
getMessages: [Message]
}
type Mutation {
postMessage(content: String!): Message
}
type Subscription {
onMessagePosted: Message
}
schema {
query: Query
mutation: Mutation
subscription: Subscription
}
Step 3: Setting Up Data Sources
Create a DynamoDB Table: In the DynamoDB console, create a table for storing messages with a primary key of id.
Add Data Source: In the AppSync console, add DynamoDB as a data source and configure resolvers for your queries, mutations, and subscriptions.
Step 4: Configuring Resolvers
Resolvers are responsible for mapping GraphQL operations to data source operations:
Query Resolver: Configure the resolver for the getMessages query to retrieve data from DynamoDB.
Mutation Resolver: Configure the resolver for the postMessage mutation to add data to DynamoDB.
Subscription Resolver: Configure the resolver for the onMessagePosted subscription to listen for new messages.
Step 5: Building the Frontend
Now that your backend is set up, it's time to build the frontend. You can use popular frameworks like React, Angular, or Vue.js with the AWS Amplify library to connect to your AppSync API.
Install AWS Amplify: In your project directory, run:
npm install aws-amplify @aws-amplify/ui-react
Configure Amplify: In your application, configure Amplify with your AppSync API details:
import Amplify from 'aws-amplify';
import awsconfig from './aws-exports';
Amplify.configure(awsconfig);
Connecting to AppSync: Use the Amplify API and PubSub modules to query data, post messages, and subscribe to real-time updates:
import { API, graphqlOperation } from 'aws-amplify';
import { listMessages, postMessage, onMessagePosted } from './graphql/queries';
// Query messages
const fetchMessages = async () => {
const messageData = await API.graphql(graphqlOperation(listMessages));
setMessages(messageData.data.listMessages.items);
};
// Post a new message
const sendMessage = async (content) => {
await API.graphql(graphqlOperation(postMessage, { input: { content } }));
};
// Subscribe to new messages
useEffect(() => {
const subscription = API.graphql(graphqlOperation(onMessagePosted)).subscribe({
next: ({ value }) => {
setMessages((prevMessages) => [...prevMessages, value.data.onMessagePosted]);
},
});
return () => subscription.unsubscribe();
}, []);
Real-World Use Cases
Use Case 1: Real-Time Chat Application
One of the most common use cases for AWS AppSync is building a real-time chat application. Users expect to send and receive messages instantly, and AppSync's subscription capabilities make this straightforward.
Message Delivery: Use subscriptions to deliver new messages to all connected clients in real-time.
Offline Support: AppSync can automatically synchronize messages when a user comes back online, ensuring no messages are lost.
Use Case 2: Collaborative Document Editing
Another powerful use case is collaborative document editing, where multiple users can edit a document simultaneously and see each other's changes in real-time.
Conflict Resolution: AppSync's conflict resolution capabilities help manage concurrent edits and ensure data consistency.
Subscriptions: Use subscriptions to broadcast changes to all collaborators in real-time.
Best Practices for Building with AWS AppSync
Schema Design: Design a GraphQL schema that reflects your application’s data requirements and operations.
Efficient Resolvers: Write efficient resolvers to minimize latency and optimize performance.
Security: Use AWS IAM, API keys, or AWS Cognito to secure your API and manage access control.
Testing and Monitoring: Regularly test your API and use monitoring tools like AWS CloudWatch to keep track of performance and errors.
Building real-time applications with AWS AppSync is both powerful and straightforward. By leveraging GraphQL, AWS managed services, and real-time subscriptions, you can create highly responsive and scalable applications. Whether you're building a chat app, a collaborative tool, or any other real-time application, AWS AppSync provides the tools you need to succeed.
References
GraphQL Basics
AWS Amplify Documentation
Disclaimer
The information provided in this blog is for educational purposes only. While every effort has been made to ensure accuracy, the author and publisher are not responsible for any errors or omissions, or for any losses or damages resulting from the use of this information. Always refer to official AWS documentation for the most up-to-date information.
Comments